High Availability in Modern Systems with distributed caching
- Published on
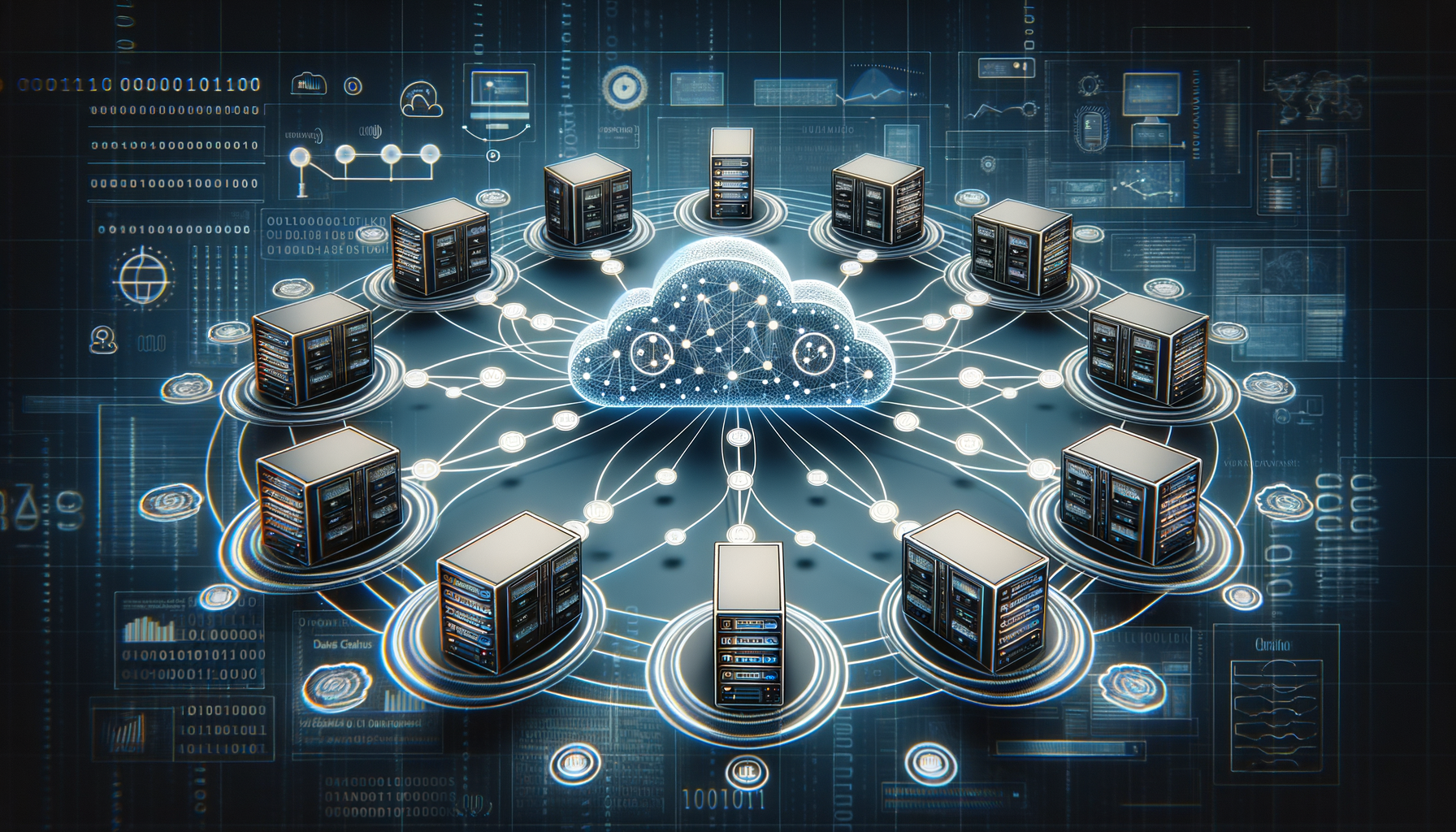
Introduction
High availability (HA) is a critical requirement for modern applications that cannot afford downtime. In essence, HA means designing systems to remain operational and responsive despite failures, often aiming for “five nines” (99.999%) or similar uptime levels (High Availability Architecture: Definition & Best Practices - Redis). One key strategy to achieve HA is leveraging distributed caching. By caching frequently used data in memory across multiple nodes, we can reduce load on primary databases and avoid single points of failure. If implemented correctly, a distributed cache ensures that even if one cache node or a backend component fails, the system can continue serving requests with minimal interruption.
Distributed caching involves spreading cached data across a cluster of servers rather than a single in-memory store. This allows the cache to scale horizontally and provides resilience. As systems grow, a single cache server becomes a liability and bottleneck. A distributed cache stored on multiple nodes means a single-node failure won’t compromise the entire cache, allowing the system to continue serving requests seamlessly (Distributed Caching: The Secret to High-Performance Applications). In other words, caching data on multiple servers provides a safety net: the application can still retrieve data from other cache nodes if one goes down, helping maintain uptime. Additionally, reducing repeated database reads by serving data from fast in-memory caches improves performance and prevents database overload, indirectly boosting overall availability.
In this article, we’ll take a deep technical dive into how distributed caching enables high availability, with a focus on Redis as the preferred technology. We will explore the concepts of distributed caching and HA, examine Redis’s architecture (replication, clustering, and failover mechanisms), and discuss the techniques Redis uses (like replication and automatic failover) to ensure fault tolerance. We’ll also cover design patterns and best practices for integrating Redis into HA systems, common pitfalls to watch out for (and how to avoid them), and real-world use cases illustrating Redis’s role in highly available architectures.
Understanding Distributed Caching and High Availability
Before delving into Redis, it’s important to understand why distributed caching is so powerful for high availability. High availability means designing systems to continue operating without interruption even when components fail (Understanding Redis High-Availability Architectures - Semaphore). In practice, this often entails redundancy and failover mechanisms: if one server or service goes down, another takes over with little or no downtime.
Caching, on the other hand, is the practice of storing frequently accessed data in a fast storage layer (like memory) so that future requests for that data can be served quicker. A distributed cache combines these ideas by storing cache data across multiple servers (or nodes). This has two major benefits for availability:
Load Reduction on Primary Datastores: By serving repeated reads from in-memory cache nodes, the cache offloads work from the primary database or API. This prevents the database from becoming a single point of failure under high load. If the database is slow or temporarily unavailable, the cache may still have the data to serve user requests, avoiding outright downtime.
Elimination of Single Cache Node Failure: In a distributed cache, data is partitioned (and often replicated) across nodes. The failure of one cache node does not mean the entire caching layer is lost. Other nodes can continue to serve at least a subset of the data, and if replication is used, the data on the failed node can be found on a replica. In contrast, a cache on a single server is a single point of failure — if that server goes down, all cached data is lost. Distributed caching inherently adds redundancy.
To illustrate, consider an application experiencing a surge in traffic. With a single cache node, that node could become overwhelmed or crash, causing a cascade of cache misses and forcing every request to hit the database (potentially crashing the database as well). With a distributed cache cluster, the load is spread out. If one node crashes or becomes inaccessible, clients can retry and fetch data from other cache nodes. The system may experience a minor performance hit, but it remains largely available to users. A distributed cache effectively “gracefully degrades” the system’s performance instead of failing completely under stress.
It’s worth noting that high availability for a cache has a slightly different goal than for a primary database. If a cache cluster goes down, the system can still function by retrieving data directly from the source of truth (albeit more slowly). The bigger risk is a cache stampede – a scenario where the cache is unavailable or a large set of data expires, and suddenly a thundering herd of requests hit the database simultaneously. This can overwhelm the database and bring down the whole system. Therefore, keeping the cache online and healthy is crucial to prevent such stampedes (Cache vs. Session Store - Redis). In summary, distributed caching contributes to HA by both reducing the likelihood of overload on critical systems and by providing an alternative data access path if the primary source is slow or down.
Now, let’s focus on Redis and see how its design enables distributed caching and high availability.
Redis Architecture and Clustering for High Availability
Redis is a leading in-memory data store renowned for its performance. But beyond raw speed, Redis provides robust features to achieve high availability: replication, automatic failover, and clustering. In a nutshell, Redis lets you run multiple servers in cooperation so that if one fails, another can take over, and it can partition data across nodes to scale out the cache size and throughput. We will examine the key architectural components of Redis HA: the leader-follower replication model, Redis Sentinel for failover, and Redis Cluster for sharding and fault tolerance.
Leader-Follower Replication in Redis
At the core of Redis’s HA strategy is leader-follower replication (also known as master-replica or primary-replica replication). In this setup, one Redis server is designated as the primary (leader) and one or more servers are replicas (followers) that continuously copy the primary’s data. The primary handles all write operations, while replicas can be used to serve read requests (read scaling) or stand by ready to take over if the primary fails (Understanding Redis High-Availability Architectures - Semaphore) (Understanding Redis High-Availability Architectures - Semaphore). By default, Redis replicas are read-only, ensuring they have an exact copy of the primary’s state at all times (Understanding Redis High-Availability Architectures - Semaphore).
(Understanding Redis High-Availability Architectures - Semaphore) Figure 1: Redis leader-follower replication. The primary node accepts writes and propagates the changes to multiple replica nodes asynchronously. Replicas acknowledge the data they receive, but the primary doesn’t wait for these acknowledgments before serving the next request, preserving Redis’s low latency characteristics (Understanding Redis High-Availability Architectures - Semaphore).
This asynchronous replication means the primary remains fast – it does not block waiting on replicas for each write operation (Understanding Redis High-Availability Architectures - Semaphore). Instead, it keeps track of how far each replica has progressed (replication offset) and sends updates in the background. The trade-off is that replicas might be slightly behind the primary. In practice, this lag is usually very small (milliseconds), but it implies that in a failover event some latest writes could be lost if the primary crashes before replicas receive them. We’ll discuss how Redis mitigates this risk (and how to design around it) later on.
Replication in Redis serves a dual purpose: data safety and high availability, and read scalability. By having copies of data on multiple servers, the system can tolerate the loss of the primary – a replica can be promoted to become the new primary, preserving the dataset. Additionally, read-heavy workloads can be spread across replicas, which not only improves throughput but also means the system is under less stress, further enhancing availability (Understanding Redis High-Availability Architectures - Semaphore). For example, expensive operations (like a large sorted set range query) can be offloaded to a replica to avoid slowing down the primary (Understanding Redis High-Availability Architectures - Semaphore). In effect, replication makes Redis a reliable distributed cache – data isn’t stored on just one volatile server, and failures can be recovered by switchover to a replica.
Redis allows a primary to have multiple replicas, even forming cascading replication chains if needed (replicas of replicas) (Redis replication | Docs). This can be useful for geo-distribution or offloading work from second-tier replicas. However, it’s most common to have a primary with a handful of direct replicas for HA. A typical deployment might be one primary with two replicas, each on separate hosts or even separate availability zones, to tolerate machine or zone failures.
One thing to note is that Redis replication is asynchronous by default. There is no built-in consensus or two-phase commit on writes – the primary will consider a write successful as soon as it’s handled locally, and replicas apply it after the fact. This maximizes performance but means a tiny window of data might not make it to the replicas if the primary fails immediately after writing. For most caching scenarios, this is an acceptable trade-off (losing a few recently cached items is usually not fatal). If stronger consistency is required, Redis provides an optional mechanism (WAIT
command or the min-replicas-to-write
configuration) to ensure a write is replicated to a certain number of replicas before considering it successful, at the cost of higher latency. Many deployments don’t enable this, relying on eventual consistency of the cache.
In summary, leader-follower replication gives Redis the foundational building block for HA: multiple copies of data on different servers. This alone enables a failover strategy — if the primary goes down, a replica can take over. Next, we’ll see how Redis implements automatic failover and role management via Redis Sentinel.
Redis Sentinel: Automated Failover and Monitoring
Having replicas is only half of the high availability story; we also need a mechanism to detect failures and promote a replica to primary automatically. This is where Redis Sentinel comes into play. Redis Sentinel is an external supervisory system that runs alongside your Redis servers to monitor them and coordinate failover when necessary (Understanding Redis High Availability: Cluster vs. Sentinel | by Praful Khandelwal | Medium).
A Sentinel deployment consists of one or (preferably) multiple sentinel processes running on separate machines (often on the same hosts as the Redis instances they monitor, but they can be separate). These processes communicate with each other and with the Redis servers to constantly check the health of the primary and replicas. In a typical HA setup, you would run at least three Sentinel instances to avoid a single point of failure in the monitoring system itself (Understanding Redis High-Availability Architectures - Semaphore) (REDIS HA with only 2 nodes).
(Understanding Redis High-Availability Architectures - Semaphore) Figure 2: Redis Sentinel architecture. In this example, we have a Redis primary with two replicas (orange boxes), and three Sentinel processes (purple) monitoring them. The Sentinels form their own small cluster (dotted lines) to exchange health information. They periodically ping the Redis servers and each other. If the primary fails or becomes unreachable, the Sentinels hold a vote to elect a leader Sentinel, which will coordinate the failover.
Redis Sentinel uses a quorum-based approach to make decisions (Understanding Redis High-Availability Architectures - Semaphore). This means a majority of the Sentinel processes must agree that the primary is down before initiating a failover. Using a quorum prevents false positives and avoids “split-brain” scenarios (where two different nodes think they are primary). For example, with three Sentinels, at least two must mark the primary as down to trigger failover (REDIS HA with only 2 nodes). This voting process ensures that network blips or a slow primary don’t cause an unnecessary failover, and that only one new primary will be chosen.
When Sentinels determine a primary is truly down, the failover sequence kicks in (Understanding Redis High-Availability Architectures - Semaphore):
One Sentinel (the leader of the election) selects the best candidate replica (based on replication offset, priority, etc.) and sends it the command to promote to primary.
The chosen replica becomes the new primary – it now accepts writes. The other replicas, if any, are reconfigured to replicate from this new primary. The old primary, if it’s still running but just cut off (e.g., network partition), will realize it’s no longer primary if it rejoins (it gets demoted to a replica to avoid split-brain).
The Sentinel cluster broadcasts the new primary’s address to any clients that ask. Sentinel acts as a service discovery provider – clients can query the Sentinels to get the current primary’s IP/port (Understanding Redis High-Availability Architectures - Semaphore). This way, after a failover, your application can reconnect to the right Redis node.
All of this happens automatically within seconds. As a result, a Redis deployment with Sentinels can provide HA: if the primary dies, you might experience a few seconds where writes are not accepted, but a replica will quickly take over and the cache service continues. Reads can even be configured to go to replicas, so they might continue uninterrupted during the failover (with the caveat that those reads might be stale until the promotion happens).
Sentinel also provides other niceties: it can send notifications (through pub/sub or scripts) to alert operators about failovers or instances going down (Understanding Redis High-Availability Architectures - Semaphore) (Understanding Redis High-Availability Architectures - Semaphore). It also manages configuration updates – for example, when a new primary is elected, the Sentinels update the Redis replicas with the new primary’s info, and also inform any Redis instances about the Sentinel cluster (so they know which Sentinels to trust). Essentially, Sentinel is the brains that turns a set of Redis instances into a coordinated, highly available cluster.
It’s important to note that clients need to be “Sentinel-aware” or use a smart Redis client library. A Redis client can connect to the Sentinels to ask, “who is the current master for this service?” and then redirect reads/writes accordingly. Many Redis client libraries support Sentinel mode out of the box. Alternatively, you can use a proxy or load balancer that is Sentinel-aware to route traffic to the current primary. The key is that after failover, the primary’s address changes, and your application must discover that. Sentinel provides the API to do so (Understanding Redis High-Availability Architectures - Semaphore).
In summary, Redis Sentinel adds fault tolerance to the replication setup by handling detection and promotion. With Sentinel, Redis can recover from the failure of the primary node automatically, without human intervention, achieving truly high availability. Next, we’ll look at Redis Cluster, which expands on these concepts by also partitioning data across multiple primaries for both HA and scalability.
Redis Cluster: Sharding and Fault Tolerance
While Sentinel + replication addresses failover, Redis Cluster tackles another aspect: scaling out the cache by partitioning data across multiple primaries, each with its own replicas. In Redis Cluster mode, the dataset is split into shards, and different nodes hold different key ranges. This allows Redis to go beyond the memory or CPU limits of a single server and handle much larger workloads, all while still providing high availability through internal replication.
A Redis Cluster typically consists of multiple primary nodes (shards), each of which can have one or more replicas. For example, you might have 3 primaries, each with 2 replicas, for a total of 9 nodes. The data is partitioned using a hashing mechanism: by default, Redis Cluster divides the key space into 16384 hash slots, and each primary is responsible for a subset of those slots (Understanding Redis High-Availability Architectures - Semaphore). When you add more shards, the slots (and their data) get redistributed across the primaries.
Each primary in the cluster replicates to its replicas just like in the leader-follower model. This means cluster mode and replication are complementary – cluster provides horizontal sharding, and replication provides redundancy within each shard. If one primary fails, one of its replicas is promoted (by the cluster itself) to take over that slot range, similarly to Sentinel’s failover.
(Understanding Redis High-Availability Architectures - Semaphore) Figure 3: Redis Cluster architecture. Here we see three shards (Shard 1, Shard 2, ..., Shard N). Each shard has a primary (yellow) and multiple replicas (orange). The cluster partitions the data (e.g., based on hash slots) such that each shard handles a portion of the keys. Replication within each shard provides HA, and the cluster as a whole coordinates which shard is responsible for which keys. Clients can connect to any node and are redirected to the right shard based on the key.
Redis Cluster uses a gossip protocol and internal messaging to coordinate the cluster. All nodes periodically communicate their status to each other (pinging to ensure others are alive, etc.), and share information about node failures or configuration changes (Redis cluster specification | Docs) (Understanding the Failover Mechanism of Redis Cluster). There’s no separate Sentinel process; the logic for failure detection and failover is built into the cluster nodes themselves. When a primary doesn’t respond, the other nodes mark it as failed (again, requiring a majority consensus of master nodes to declare a failure) and will promote a replica if one is available (Understanding Redis High-Availability Architectures - Semaphore). This means Redis Cluster can self-heal when a node dies: it recomputes which nodes are primaries, and updates the cluster state accordingly. The client will then be redirected to the new primary for that shard.
From a high-availability standpoint, Redis Cluster ensures that the cache service remains online even if some subset of nodes fails or becomes unreachable (Understanding Redis High-Availability Architectures - Semaphore). For example, if you have a cluster with 6 primary nodes (shards) and each has one replica, the cluster can tolerate up to 6 failures (provided no shard loses all its nodes). If one primary goes down, its replica takes over that hash slot range, and the rest of the cluster is unaffected – so most of your cache is still available, and the affected shard’s data is still served by the new primary. Clients might see a brief interruption for keys in the failed shard during the promotion process, but overall the cluster continues to operate (Understanding Redis High-Availability Architectures - Semaphore).
Redis Cluster not only gives HA through replication, but also scales out throughput and memory capacity by using multiple primaries. Applications that need very large caches (beyond one server’s RAM) or extremely high throughput (beyond one server’s CPU/network) can use cluster mode to distribute the load. This is crucial for high availability because it avoids the scenario where a single busy cache server becomes a choke point that could fail under load. Instead, load is balanced. Furthermore, cluster mode isolates failures to some extent: if one shard’s data has an issue, it doesn’t directly impact the others. The cluster can also be configured to survive network splits by requiring a majority of masters to be online to continue operations, thereby avoiding split-brain conditions.
One must be aware, however, that Redis Cluster introduces some constraints. Multi-key operations (like a transaction involving multiple keys) only work if the keys are on the same shard (sharing the same hash slot). There are ways to ensure related keys go to the same shard (using key hash tags), but developers need to design with data partitioning in mind (Understanding Redis High-Availability Architectures - Semaphore) (Understanding Redis High-Availability Architectures - Semaphore). This is usually not a big issue for caching use cases, but it’s a consideration when integrating Redis Cluster into your system.
Cluster vs Sentinel: Redis offers both approaches – you can use Sentinel with a single primary (and replicas) for HA, or you can use Cluster which inherently uses multiple primaries (with replicas). Sentinel is simpler and is often used when your dataset fits on one machine but you want failover. Cluster is used when you need to scale beyond one machine and still want HA. It’s possible to combine them (Cluster with replicas is essentially using the cluster’s internal Sentinel-like mechanism for each shard). Many cloud providers and enterprise setups use cluster mode for large-scale systems.
In practice, setting up Redis Cluster requires at least 3 primary nodes (shards) for a minimal viable cluster, and typically each with at least one replica for HA. That’s why you often see recommendations of at least 6 nodes (3 primaries + 3 replicas) so that no primary is without a backup and no two copies of a shard reside on the same physical machine (Understanding Redis High Availability: Cluster vs. Sentinel | by Praful Khandelwal | Medium). This ensures that if a machine or VM goes down, it doesn’t take out a shard completely. For smaller use cases, a Sentinel-based setup (one primary, two replicas) on 3 machines might suffice. The choice depends on data size and throughput needs.
To sum up, Redis Cluster provides an HA solution that also delivers horizontal scaling. It partitions data across nodes and uses replication and automatic failover within each partition to keep the service running through failures. Operations can continue even if a subset of nodes fails or is isolated, which means higher reliability and fault tolerance for your caching layer (Understanding Redis High-Availability Architectures - Semaphore).
Having covered how Redis’s architecture supports high availability through replication, sentinel, and clustering, we’ll now discuss best practices and design patterns for integrating Redis into your systems to maximize uptime.
Best Practices and Design Patterns for Using Redis in Highly Available Systems
Achieving high availability isn’t just about the core technology features (replication, failover) — it also involves how you integrate Redis into your application architecture and how you configure and operate it. In this section, we cover design patterns for caching with Redis and best practices to ensure the caching layer itself is resilient and reliable.
Caching Patterns for High Availability
When using Redis as a cache alongside a primary database, it’s important to choose the right caching pattern to balance performance, consistency, and failure behavior. The main caching patterns are cache-aside, write-through, and (less commonly) write-behind (also known as write-back). Each has implications for availability:
Cache-Aside (Lazy Loading): This is the most common pattern. The application checks the cache first on a read; if the data is found (cache hit), great – it’s returned quickly. If not (cache miss), the application fetches from the database, then populates the cache with that data for next time (Caching patterns - Database Caching Strategies Using Redis) (Caching patterns - Database Caching Strategies Using Redis). Writes go directly to the database, and optionally the cache is updated (or simply evicted) afterward. This pattern is simple and keeps the cache in sync with actual usage – only data that is needed is cached. It also means that if the cache cluster goes down or is flushed, the system still works (all reads go to the DB, albeit slower). This fail-safe behavior is good for availability: the cache is an accelerator, not a source of truth. After a failure, the cache can gradually warm up again as data is requested. The downside is the first request for an item incurs a higher latency (and if the cache is cold, many misses can flood the database – this is the cache stampede risk). Still, for HA it’s beneficial that a cache-aside setup gracefully handles cache failures by falling back to the database. Most systems use cache-aside by default (Caching patterns - Database Caching Strategies Using Redis).
Write-Through: In this pattern, every time the database is updated, the cache is proactively updated as well (Caching patterns - Database Caching Strategies Using Redis). For example, after writing a record to the DB, the application immediately writes the same data to Redis (or even writes to Redis first and let a background process update the DB). This ensures the cache is always up-to-date with the latest data, avoiding cache misses for recently written data (Caching Best Practices | Amazon Web Services) (Caching Best Practices | Amazon Web Services). The advantage for availability is that users are less likely to ever hit the slower database path – even new or recently changed data is in the cache, so the system stays speedy and under less load. However, a potential downside is that if the cache cluster is temporarily unavailable, your writes might fail (or you have to queue them) since the application expects to update cache on every operation. Also, a write-through cache can accumulate a lot of data that might never be read (unnecessary utilization) (Caching Best Practices | Amazon Web Services). Many systems use a combination: cache-aside as a baseline, and write-through for certain hot data that you know will be read frequently, to preemptively cache it (Caching Best Practices | Amazon Web Services). If a write-through cache node fails, it behaves similarly to cache-aside – the data is still safely in the DB, and the cache will miss until repopulated.
Write-Behind (Write-Back): In this less common pattern, the application writes only to the cache, and the cache later syncs those changes to the database asynchronously. This can improve write latency (the user isn’t waiting on the database), but it introduces complexity and risk. If a cache node fails before the write is flushed to the DB, that data might be lost. Most use cases that need this level of performance and can tolerate some data loss would actually treat Redis as the primary store (with persistence) rather than an ephemeral cache. For high availability, pure write-behind is risky unless you have robust measures to recover any lost writes (such as Redis persistence/AOF enabled, or a stable queue of pending writes). In practice, if using write-behind, you’d still want replication and maybe persistence on Redis to avoid losing the buffered writes. This pattern is usually avoided for critical data.
In general, cache-aside is recommended for most scenarios because it provides a good balance: the system can survive without the cache (just slower), making it inherently more fault-tolerant. Write-through can be added to reduce miss penalties in specific cases. Both patterns keep the database as the source of truth, which means a cache failure is not catastrophic to data integrity. As noted, whichever pattern you use, be mindful of the cache stampede issue: if the cache is cold or fails, many requests may hit the DB at once. Techniques such as request coalescing (only let one thread load a missing key while others wait), or staggering expiration times on cache keys, can mitigate this. The goal is to ensure that when the cache is recovering (cold start after failure), it doesn’t overwhelm the backend – thereby maintaining overall system availability.
Deployment and Configuration Best Practices
Beyond how your application uses the cache, there are several best practices in deploying and configuring Redis for high availability:
Use Multiple Availability Zones or Hosts: Don’t put all Redis nodes on the same physical server or in the same failure domain. If you have replicas, ensure the primary and its replicas are on separate machines or AZs. This way, a hardware failure or network outage in one zone doesn’t wipe out all copies of your data. Cloud managed Redis services (AWS ElastiCache, Azure Cache for Redis, etc.) typically offer multi-AZ deployments for exactly this reason – e.g., a primary in one zone and a replica in another, giving automatic failover across zones (often yielding 99.99% availability SLA) (High availability for Azure Managed Redis (preview) - Azure Managed Redis | Microsoft Learn) (High availability for Azure Managed Redis (preview) - Azure Managed Redis | Microsoft Learn).
At Least Three Sentinels (or Odd Quorum) in Production: If you are using Redis Sentinel for HA, always deploy an odd number of Sentinel processes (with a minimum of three). This ensures a majority vote is possible if one node goes down (REDIS HA with only 2 nodes). With only two Sentinels, for example, a single failure means the remaining one can’t form a quorum alone to elect a new master. The same idea goes for Redis Cluster – have an odd number of master nodes if possible, and ensure a majority of masters must be up for the cluster to be functional, to avoid split-brain situations. Essentially, quorum-based systems need quorum nodes! Even if you only have two data nodes, you might run a third Sentinel on a tiny instance just as a tiebreaker (even a Raspberry Pi, as one Redis maintainer jokingly suggested (REDIS HA with only 2 nodes)).
Configure Persistence for Critical Data or Use AOF: By default, Redis is an in-memory store that can use optional persistence (RDB snapshots, AOF logs) to save data to disk. In pure caching scenarios, losing the data might be acceptable (since the source of truth is elsewhere). But if your cached data is critical to instantly recover (e.g., session store, or simply to avoid a massive cache stampede on restart), consider enabling RDB snapshots or AOF persistence. At the very least, enable it on one replica. A common pattern is to have the primary not persist to avoid any pause, and a replica that does persist to disk periodically (Redis replication | Docs). However, be cautious: if you disable persistence on the primary and it restarts, it will come up empty and replicas will sync this empty state, erasing all cached data on replicas too (Redis replication | Docs). This is a well-known pitfall. To avoid it, if you turn off persistence on the primary for performance, either disable automatic restart for that instance or ensure the process does not start before a failover can happen (Redis replication | Docs). In general, for HA, having persistence on at least some nodes is good practice — it allows faster restarts and can act as a fallback if all nodes fail (you can recover the last snapshot). For purely ephemeral caches, you might accept losing data, but be aware of the stampede effect on recovery.
Avoid Co-locating Master and Replica on One Host: This applies to cluster setups: ensure that a single physical machine or VM does not contain both a primary and its own replica. Otherwise, if that host dies, it takes out both copies of one shard. Redis Cluster doesn’t automatically ensure this (it randomizes assignment unless you specify a preferred layout). When using cluster, use the topology configuration to assign primaries and replicas to distinct availability groups. A best practice is the “6 nodes (3 primary + 3 replica) on 3 hosts” rule – this way, even if one host goes down, each of the 3 primaries has a replica on a different host to take over (Understanding Redis High Availability: Cluster vs. Sentinel | by Praful Khandelwal | Medium).
Tune Timeouts and Client Retry Logic: From the client perspective, make sure your application has appropriate timeouts when talking to Redis and implements retry logic for transient errors. If a failover is in progress, clients may see a few “connection refused” or “movable” errors. Well-written client libraries will handle this (for example, Redis Cluster clients will follow redirections to the new node; Sentinel-aware clients will reconnect to the new master). Ensure your app doesn’t crash or give up immediately on a single failure – a short retry (with backoff) can bridge the gap during failovers. This makes the system more resilient to brief unavailability of the cache.
Use Connection Pooling and Avoid Exhausting Resources: Make sure to reuse Redis connections or use a connection pool. Creating new connections for every request can overwhelm the Redis server (many small ephemeral connections) and also the system’s file descriptors. This is more of a performance best practice, but an overwhelmed Redis could become unresponsive (affecting availability). Many languages have libraries that manage a pool of Redis connections.
Set Memory Eviction Policy and Monitor Memory: Redis is in-memory, so you should configure an eviction policy (like LRU – Least Recently Used) if there’s any chance of reaching memory limits. This way Redis will start evicting old data when full rather than crashing or refusing writes. For caches, an eviction policy is essential – usually an LRU or LFU policy is chosen so that the least-used items get dropped first to free space. Also consider setting a maximum memory usage (
maxmemory
config) and apply TTLs (time-to-live) on cache entries. In fact, always set a TTL on your cache keys (except perhaps truly static data) – eventually expiring entries prevents stale data from living forever and clears out keys that the app might forget to invalidate (Caching Best Practices | Amazon Web Services). If you never expire anything, your cache could fill up and then evict things in a unpredictable manner or hit memory errors. Using TTLs (even long ones, like hours or days) ensures that if your app forgets to invalidate a cache entry when the underlying data changes, the stale value won’t stick around forever (Caching Best Practices | Amazon Web Services). It also guards against memory leaks in the caching layer.Monitor and Alert: Treat your cache cluster as a critical part of the infrastructure – monitor its health and performance. Key metrics to watch include memory usage, eviction counts, hit/miss rates, replication lag, and failover events. Monitoring replication lag is particularly important in HA setups; if a replica falls too far behind the primary, it might not be able to fail over without data loss. Redis provides info statistics and commands to check these. Set up alerts for conditions like “replication link down” or “memory usage above X%” so you can act before it impacts availability.
Plan for Capacity (N+1 Nodes): When sizing a Redis cluster, account for the failure scenario. For example, if you have 3 shards each on separate nodes with one replica each, can the remaining nodes handle the entire load if one fails? In cluster mode, if a primary fails, its replica takes over – but now you have one less replica until you add a new one. If another failure happens before you restore redundancy, you could lose data. So, it’s wise to run with more capacity than needed (an extra node or the ability to spin up a replacement quickly) and possibly an extra replica in critical shards. In cloud environments, use autoscaling or have “cold standby” nodes that can be promoted. The idea is to avoid running at the edge of capacity, so that failover events don’t overload the surviving nodes.
By following these best practices, you create a robust Redis caching tier that can withstand common failure modes. However, even with the best design, there are pitfalls that teams sometimes encounter. In the next section, we’ll discuss some of those common pitfalls and how to avoid them.
Common Pitfalls and How to Avoid Them
Even experienced engineers can run into issues when operating a distributed cache. Here are some common pitfalls in using Redis for high availability, and ways to mitigate them:
Not Enough Sentinels or Quorum Nodes: As mentioned, running only 1 or 2 Sentinel instances is a mistake – it can prevent automatic failover. Avoid: Always run a quorum of Sentinels (3 or 5). Similarly, in a cluster, remember that a majority of master nodes must be active; if you partition your cluster in half, neither side will have quorum and the cache may become unavailable. Design your deployment to favor one side (odd number of primaries).
Primary and Replica on the Same Server: A very common misconfiguration is running a Redis primary and its replica on the same host (or VM/hypervisor). This might happen inadvertently in virtualization or if not carefully placing instances. If that host goes down, you lost both the primary and replica in one blow – meaning data is lost until recovery. Avoid: As a rule, ensure redundancy by placing replicas on different physical machines or fault domains than their master (Understanding Redis High Availability: Cluster vs. Sentinel | by Praful Khandelwal | Medium). Many orchestration tools (like Kubernetes operators or Terraform scripts) can enforce anti-affinity for Redis instances.
Assuming Data Is Safe with Replication But Disabling Persistence Incorrectly: Some assume that because Redis has replicas, you don’t need disk persistence. However, as outlined earlier, if the primary restarts empty and your replicas sync to it, you can wipe all copies of data (Redis replication | Docs). Avoid: Either enable RDB/AOF persistence on primaries (so they don’t restart empty), or if you must disable it, ensure that a crashed primary doesn’t auto-restart before you promote a replica. One configuration is to turn off
autorestart
in systemd or Docker for the Redis container, so that if it crashes, it stays down and Sentinel will do a proper failover instead of the primary coming back up alone. Always test these failure scenarios in staging to be sure your setup does the right thing.Split Brain in Failover: A split brain is when two Redis instances both think they are the primary. This can happen if the network partitions and the Sentinels (or cluster nodes) don’t agree on who is primary. You might end up with the original primary still accepting writes in one partition and a new primary in another partition. When the network heals, data divergence is a problem. Avoid: Using quorum and Sentinel’s design usually prevents this (majority required for failover). To further reduce risk, you can configure
min-replicas-to-write
on the primary – for example, require at least 1 replica connected and up-to-date, otherwise the primary will stop accepting writes (REDIS HA with only 2 nodes). This way, if a primary gets isolated from all replicas (and presumably from Sentinels), it will refuse writes, reducing split-brain impact (it essentially “sacrifices” availability to preserve consistency in that edge case). Also, if using cluster mode, setting the cluster to pause if too many primaries are down (cluster require-full-coverage config) can prevent serving possibly inconsistent data. Monitoring and quickly detecting network partitions is key too.Cache Stampede (Cold Cache) After Failure: If your entire Redis cluster goes down and restarts empty (or you fail over to an empty new node because none of the replicas survived), your application might suddenly slam the database as it rebuilds the cache. This stampede can cause a secondary outage of the database. Avoid: Implement protections for cache stampede. Tactics include request collapsing (only allow one thread or a limited number of threads to fetch a missing key while others wait), graceful degradation (serve users a possibly stale page or a maintenance message for non-critical parts while the cache warms up), or rate-limiting the cache misses. Also staggering TTLs so that not everything expires at once helps – don’t have all keys set to expire on the hour, for instance. Some advanced strategies use a two-tier cache: local in-process caches that can serve during a Redis outage for a short time, or a fallback read of slightly stale data from a replica that wasn’t promoted, etc. The main point is to be aware of the thundering herd problem and have a plan to mitigate it, thereby keeping the system up.
Inefficient Client Configuration Leading to Timeouts: A subtle pitfall is not configuring clients properly in the event of failover. For example, a client might by default retry a lost connection aggressively or wait too long. If you have many clients all with a long blocking timeout, when a failover happens they might all hang and then all retry at once, causing a spike. Avoid: Tune client library settings for your scenario. Ideally, use client libraries that support targeted failover handling (like Redis Cluster clients that handle MOVED/ASK redirections, and Sentinel clients that auto-discover the new master). Set sane socket timeouts so that operations error out quickly if Redis is down, allowing your code to implement its own retries or fallbacks.
Security Misconfigurations Affecting Availability: Sometimes in trying to achieve HA, people open up Redis too broadly (like binding it to 0.0.0.0 with no firewall for convenience). This can lead to malicious actors flushing your cache or overloading it (there have been real incidents of exposed Redis servers being attacked). A compromised cache is an availability threat (not to mention data breach). Avoid: Always secure Redis – if it’s within a VPC or internal network, still use firewall rules or at least require authentication (
requirepass
or ACLs in Redis). Never assume “it’s just a cache, it’s fine if public”. An attacker could delete all keys or fill the memory, causing your service to crash or become slow.Ignoring Resource Limits or System Bottlenecks: Ensure the machine hosting Redis has reliable resources. E.g., if the OS overcommits memory and starts swapping, Redis performance will plummet (an in-memory DB swapping to disk is extremely slow) and it may appear “down.” Similarly, hitting file descriptor limits or bandwidth limits can make Redis unreachable. Avoid: Monitor system-level metrics too. Disable swapping for Redis servers (or use
memlock
/noeviction
carefully), setovercommit_memory=1
if using Linux with large memory, and make sure to allow enough file descriptors (ulimit
) for Redis to handle all client connections.
By anticipating these pitfalls and applying the recommended practices, you can greatly improve the resilience of your Redis caching layer. Many of these lessons have been learned the hard way by others, so building in these safeguards from the start will save you downtime.
Real-World Use Cases of Redis in Highly Available Architectures
Redis’s capabilities for high availability and performance have made it a staple in the tech stacks of many companies. Here are a few real-world scenarios and case studies that demonstrate how Redis is used to ensure systems remain highly available:
Large-Scale Web Applications and E-Commerce: Online retailers and large web platforms use Redis to cache product catalogs, user profiles, and session data to handle massive traffic spikes (like Black Friday sales) without crashing their databases. For example, Freshworks (a SaaS company) experienced enormous growth that strained their primary MySQL database; by introducing Redis as a caching layer, they reduced the load on MySQL and improved response times without compromising availability (Top 5 Redis Use Cases | Redis). The Redis layer ensured that read traffic could be served even if the SQL database was under heavy load, thereby keeping the application responsive. Many e-commerce sites also use Redis for user sessions and shopping cart data – this allows a user’s session to survive an app server failure (since any server can fetch session info from Redis) and keeps the site running smoothly even if parts of the infrastructure have issues.
Social Media and Gaming (Real-Time Data): Redis is popular in social networks and online games where real-time leaderboards, feed timelines, or counters are needed. These systems require low latency and also need to be up 24/7. Companies like Twitter have used caching systems (similar to Redis) to store timelines, and many gaming companies use Redis for storing game state or high-score tables. The high availability comes from Redis Cluster distributing the data and ensuring replicas are ready if a node fails. For instance, a global leaderboard updated by millions of players can be sharded across a Redis Cluster. If one node goes down, that portion of the leaderboard might briefly freeze, but a replica takes over and players can continue to see and update scores. The rest of the game services remain unaffected. Redis’s ability to handle Pub/Sub and stream data is also leveraged for real-time chat in games or social apps, often with replication so that if a chat node fails, another takes over the channel.
Session Stores and Authentication: Many web applications externalize session storage to Redis so that the application servers can be stateless (which helps with HA for the app tier). In these cases, Redis itself must be highly available, because if Redis is down, no user can log in or maintain a session. By using Sentinel or Cluster, companies ensure the session store Redis is redundant. As an example, an authentication microservice might use Redis (with replication) to store login tokens or rate-limiting counters. If one Redis instance fails, the service can quickly fall back to the replica, so logins continue without downtime. This pattern is common in cloud services and APIs – for example, GitHub and Stack Overflow have used Redis for session and cache data to handle millions of users. The key is that any single web server can fail (since session state is in Redis), and even Redis can fail and recover via failover, without logging users out (Cache vs. Session Store - Redis).
Microservices and Distributed Systems: Redis is often deployed as a shared caching layer or message broker in microservices architectures to ensure data consistency and availability across services. For instance, in a microservices-based e-commerce system, one service might cache inventory counts in Redis so that multiple frontend services can quickly check stock without hitting the central database. If the inventory database goes down, the cached values in Redis can still allow the site to display approximate stock levels (maybe with a warning) rather than failing completely. Additionally, features like Redis Streams or Pub/Sub are used for inter-service communication with high availability – if one Redis node fails, a replica continues the stream so that no messages are lost and services continue to communicate.
Cloud Managed Services: Many companies opt for managed Redis services (like Amazon ElastiCache for Redis or Azure Cache for Redis) which inherently provide high availability setups. These services typically use Redis under the hood with multi-AZ replication and automated failover. For example, Azure’s managed Redis offers a 99.9% SLA for a standard two-node (primary/replica) setup, and 99.99% for zone-redundant clusters (High availability for Azure Managed Redis (preview) - Azure Managed Redis | Microsoft Learn) (High availability for Azure Managed Redis (preview) - Azure Managed Redis | Microsoft Learn). This shows in practice that a well-configured Redis can achieve very high uptime. Cloud providers have numerous case studies of customers who rely on these services to keep their applications always-on. The benefit of managed solutions is that a lot of the heavy lifting of failover and monitoring is handled for you, but the architectural principles remain the same.
Each of these use cases underscores a common theme: Redis helps maintain both performance and availability under heavy load or failure conditions. By caching critical data and providing fast failover, it allows the overall system to stay responsive and available to users. Whether it’s serving a popular social feed, keeping an online store running through a database outage, or scaling an enterprise SaaS product to millions of users, Redis’s high availability features are often a foundational component.
Conclusion
Distributed caching with Redis has proven to be a game-changer in building highly available systems. By storing data in memory across multiple nodes, Redis dramatically reduces access latency and shields backend databases from load spikes, all while providing mechanisms to avoid downtime. Through asynchronous replication and careful failover orchestration, a Redis deployment can continue serving data even when individual servers crash. Techniques like Redis Sentinel and Redis Cluster ensure there is no single point of failure: if one node goes down, another steps in to keep the cache (and thus the application) running.
In this article, we explored how distributed caching contributes to high availability, and dug into Redis’s architecture – covering replication, Sentinel, and clustering – that makes such resilience possible. We discussed design patterns like cache-aside and write-through caching that influence the behavior during failures, and we highlighted best practices (from using multiple AZs to setting proper TTLs) to get the most HA out of a Redis setup. We also went over pitfalls to avoid, so that your high availability configuration doesn’t inadvertently become a vulnerability (for example, a misconfigured replica or an unmonitored failover).
The real-world examples illustrate that these aren’t just theoretical ideas; companies large and small rely on Redis to stay online in the face of traffic surges and server outages. Redis has matured over the years to offer a robust suite of HA features – including recent advancements like Redis Enterprise’s active-active geo-replication for multi-region HA, should your needs extend that far (High Availability Architecture: Definition & Best Practices - Redis).
In conclusion, Redis, when used thoughtfully, can greatly increase the resilience of your infrastructure. It provides the speed needed for performance and the redundancy needed for availability. By integrating a distributed Redis cache into your architecture, you enable your applications to deliver fast, reliable service to users at all times – even when the unexpected happens. High availability is a multi-layered challenge, but Redis is a proven tool in the engineer’s arsenal to meet that challenge, ensuring that data is always at hand when and where it’s needed.