Unlocking the Power of Python's Generators
- Published on
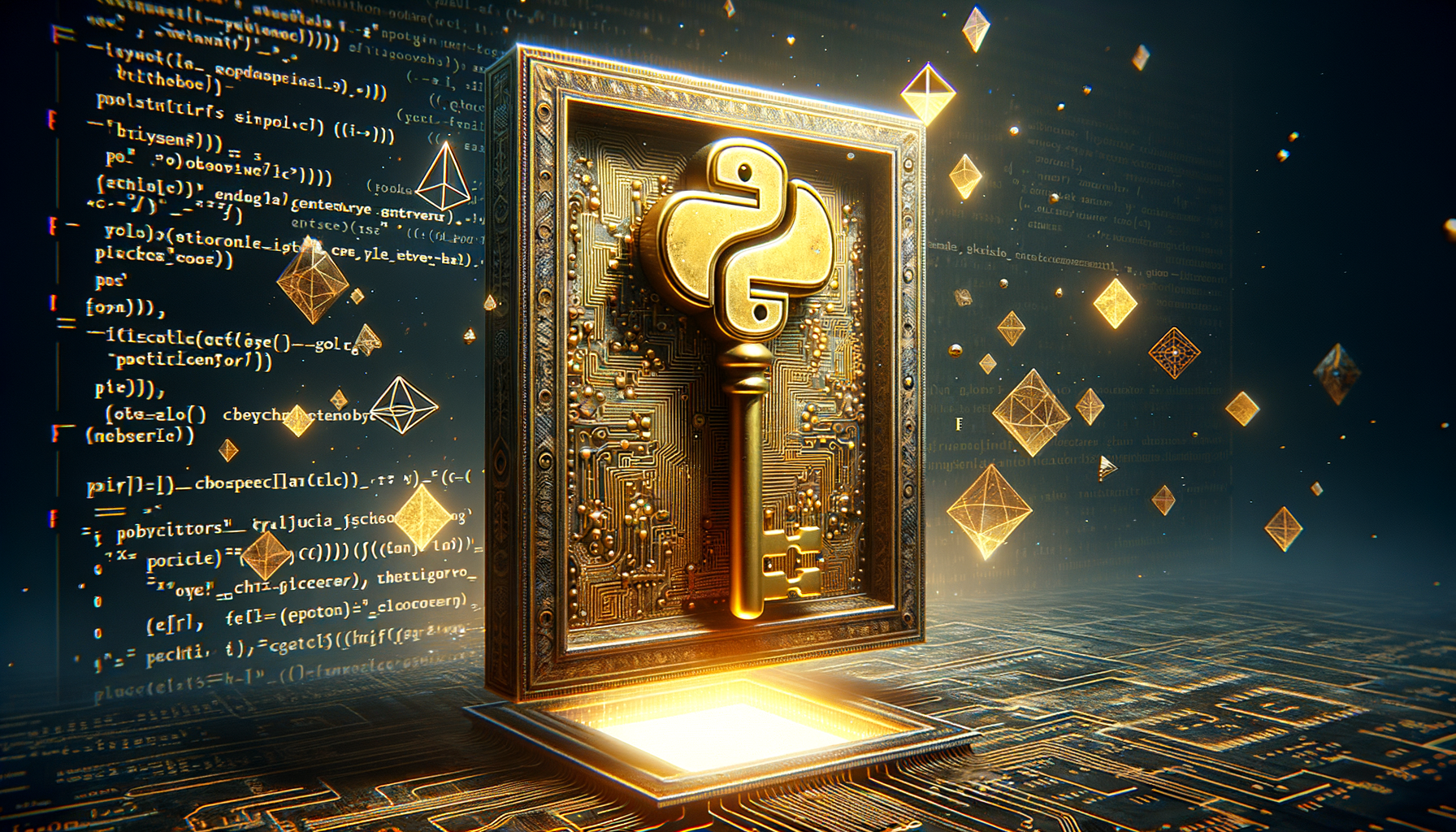
As developers, we're always on the lookout for tools that make our code more efficient and readable. Python's generators are one such feature that can significantly enhance the way we handle data processing. In this article, we'll dive into what generators are, why they're useful, and how you can incorporate them into your Python projects.
What Are Generators?
Generators are a special class of iterators in Python that allow you to iterate over data without storing it entirely in memory. Unlike lists or tuples, generators produce items one at a time and only when required. This "lazy evaluation" makes them incredibly memory-efficient, especially when dealing with large datasets.
Why Use Generators?
- Memory Efficiency: Since generators yield one item at a time, they are ideal for processing large files or streams of data without loading everything into memory.
- Improved Performance: Generators can lead to faster execution times because they compute values on the fly.
- Clean Syntax: They help in writing concise and readable code, making your data processing pipelines easier to understand and maintain.
Creating Generators
1. Generator Functions with yield
A generator function looks like a normal function but uses the yield
statement to return data:
def count_up_to(max_value):
count = 1
while count <= max_value:
yield count
count += 1
Usage:
counter = count_up_to(5)
for num in counter:
print(num)
Output:
1
2
3
4
5
2. Generator Expressions
Generator expressions are similar to list comprehensions but use parentheses instead of square brackets:
squares = (x * x for x in range(10))
for square in squares:
print(square)
Practical Examples
Reading Large Files
def read_large_file(file_path):
with open(file_path, 'r') as file:
for line in file:
yield line.strip()
for line in read_large_file('large_log_file.txt'):
process(line)
Infinite Sequences
def infinite_sequence():
num = 0
while True:
yield num
num += 1
for number in infinite_sequence():
if number > 100:
break
print(number)
Chaining Generators
You can chain multiple generators together to create a data processing pipeline:
def generate_numbers(n):
for i in range(n):
yield i
def filter_even(numbers):
for num in numbers:
if num % 2 == 0:
yield num
def square(numbers):
for num in numbers:
yield num * num
numbers = generate_numbers(10)
even_numbers = filter_even(numbers)
squared_numbers = square(even_numbers)
print(list(squared_numbers))
Output:
[0, 4, 16, 36, 64]
Conclusion
Generators are a powerful feature that can make your Python code more efficient and elegant. They are perfect for handling large datasets, implementing pipelines, or any scenario where you want to conserve memory. By incorporating generators into your toolkit, you can write cleaner code that's both performant and easy to maintain.